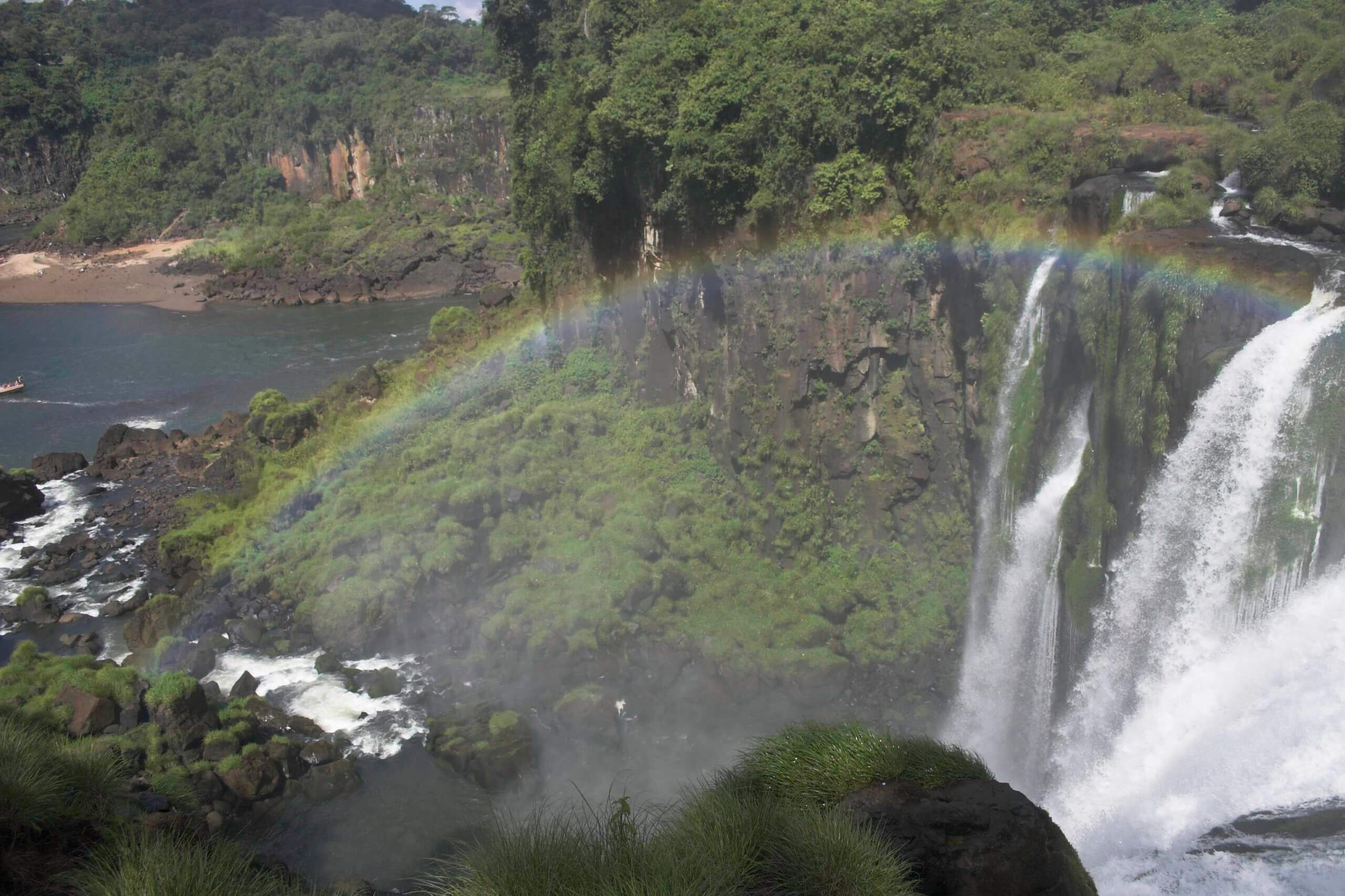
I was recently working on a project that required I setup a VAST/VPAID waterfall within VideoJS, and pass macros to be replaced on runtime to the ad tag. It was pretty tricky to get it working, but after much research, I was able to accomplish it.
Here’s how I did it.
Setting up VideoJS
I first followed the instructions to setup VideoJS, plain vanilla, stock, just as-is and nothing fancy. Made sure that was working.
I found this hosted mp4 video which is great for testing if you don’t want to eat up your own bandwidth.
Setting up VideoJS Contrib Ads
Setting up Google’s VideoJS IMA PluginI downloaded and installed the two /src files that came with it.
[sourcecode]<link href="/vjs/videojs.ima.css" rel="stylesheet">
</head>
…
<script src="//imasdk.googleapis.com/js/sdkloader/ima3.js"></script>
<script src="/vjs/videojs.ima.js"></script> [/sourcecode]
You’ll need to add the ima3.js file from Google as well, which you can see above.
VideoJS IMA suggests adding a player.js with the config and referencing that, which I’ve done and it worked quite well. Here’s what my player.js file looks like.
[sourcecode]var height = getComputedStyle(document.getElementById(‘content_video’)).height;
var width = getComputedStyle(document.getElementById(‘content_video’)).width;
var pageurl = encodeURIComponent(window.location);
var referrer = encodeURIComponent(document.referrer);
var options = {
id: ‘content_video’,
adTagUrl: "http://your-server.com/vast.php?h="+height+"&w="+width+"&pageurl="+pageurl+"&referrer="+referrer
};
player.on(‘adserror’, function(){
// do something on adserror
});
player.ima(options);
player.ima.requestAds();
player.play();
[/sourcecode]
You will notice that I’m passing four different parameters from javascript to the PHP file and I’m doing this because XML doesn’t natively support macros, nor does this setup — from what I researched. So I decided to make my own macro system because many of the third-party tags I’m using, required macros to be replaced on runtime.
Setting up vast.php
The next step is to setup your VAST tag with your ad networks.
[sourcecode]<?php header("Content-Type: application/xml; charset=utf-8"); ?>
<?php echo "<?xml version=\"1.0\" encoding=\"UTF-8\"?>\n\n"; ?>
<?php
$page_url = urldecode($_GET[‘pageurl’]);
$referrer = urldecode($_GET[‘referrer’]);
$width = $_GET[‘w’];
$height = $_GET[‘h’];
?>
<VAST version="2.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="vast.xsd">
<Ad id="1">
<Wrapper>
<AdSystem>ADSERVER</AdSystem>
<VASTAdTagURI>
<![CDATA[http://AD_TAG_GOES_HERE?&playerWidth=<?php echo $width; ?>&playerHeight=<?php echo $height; ?>&playerPosition=1&srcPageUrl=<?php echo $page_url; ?>]]>
</VASTAdTagURI>
<Extensions>
<Extension type="waterfall" fallback_index="0" />
</Extensions>
</Wrapper>
</Ad>
<Ad id="2">
<Wrapper>
<AdSystem>xy</AdSystem>
<VASTAdTagURI>
<![CDATA[http://AD_TAG_GOES_HERE?playerWidth=<?php echo $width; ?>&h=<?php echo $height; ?>&url=<?php echo $page_url; ?>&cb=<?php echo rand(); ?>]]>
</VASTAdTagURI>
<Extensions>
<Extension type="waterfall" fallback_index="1" />
</Extensions>
</Wrapper>
</Ad>
</VAST>[/sourcecode]
CRITICAL STEP – CORS
I ran around in circles while trying to figure this one out, hopefully I’ll save you some time.
You need to make sure to allow for CORS headers on the vast.php file for this to work right. Add the following to the .htaccess file in the same folder (or parent) of your vast.php file for it to work.
[sourcecode]Header add Access-Control-Allow-Origin "*"
Header add Access-Control-Allow-Headers "origin, x-requested-with, content-type"
Header add Access-Control-Allow-Methods "PUT, GET, POST, DELETE, OPTIONS"[/sourcecode]
Wrapping it up…
After throwing all of this into a single file, here’s what my index.html page looks like.
[sourcecode]<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<title>Video.js Text Descriptions, Chapters & Captions Example</title>
<link href="/video-js.min.css" rel="stylesheet">
<link href="/videojs.ads.css" rel="stylesheet">
<link href="/videojs.ima.css" rel="stylesheet">
</head>
<body>
<video id="content_video" class="video-js vjs-default-skin" controls autoplay preload="auto" width="768" height="432" >
<source src="http://rmcdn.2mdn.net/Demo/vast_inspector/android.mp4" type="video/mp4" />
</video>
<script src="/video.js"></script>
<script src="/videojs.ads.min.js"></script>
<script src="//imasdk.googleapis.com/js/sdkloader/ima3.js"></script>
<script src="/videojs.ima.js"></script>
<script src="/player.js"></script>
</body>
</html>[/sourcecode]
Load up your own tags, test the page and watch the network console, and you should be good to go! Please feel free to tear apart the code, I’m still a pretty early novice, but I enjoy programming.
Reference URLs
- VideoJS – http://videojs.com/
- VideoJS Contrib Ads – https://github.com/videojs/videojs-contrib-ads
- Google’s IMA VAST Plugin – https://github.com/googleads/videojs-ima
- Tremor’s VAST Inspector – http://tagvalidator.videohub.tv
- And some great feedback from Shawn Busolits – https://github.com/shawnbuso – thanks Shawn!